Introduction to Structured and Modular Programming
Structured Programming and Modular Programming are two key approaches in software development that help improve code readability, maintainability, and efficiency.
Structured Programming
Structured programming is a programming paradigm that emphasizes a clear, logical structure by using sequences, selection (if-else, switch), and iteration (loops). It avoids the use of goto statements, making programs easier to read and debug.
A programming paradigm is a fundamental style or approach to programming that provides a way to structure and organize code. It defines the principles, techniques, and logic used in writing programs.
In the case of Structured Programming, it is a programming paradigm that emphasizes:
✔ A clear and logical flow of control.
✔ The use of sequences, selection (if-else, switch), and iteration (loops).
✔ Avoiding goto statements to improve readability and maintainability.
Other common programming paradigms include:
- Procedural Programming (e.g., C)
- Object-Oriented Programming (OOP) (e.g., C++, Java)
- Functional Programming (e.g., Haskell, Lisp)
goto
statements are commands in programming that allow the program to jump to a specific label in the code. However, they are generally discouraged because they can make the code harder to read, debug, and maintain.
Example of goto
in C:
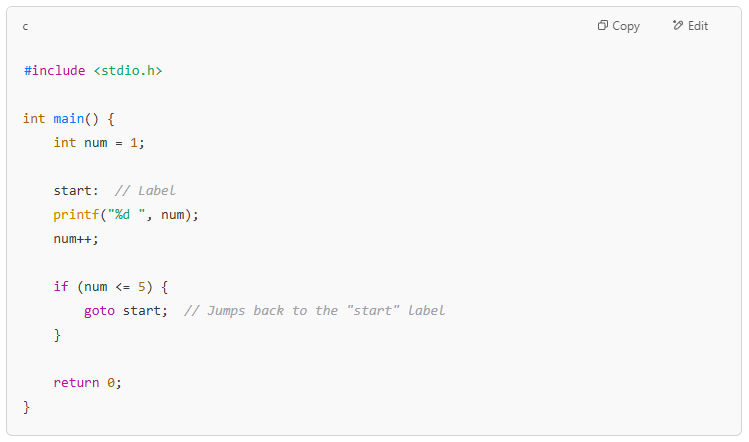
🔴 This code uses goto
to create a loop, but it’s not recommended because it makes the flow less structured.
Better Alternative (Using a Loop):
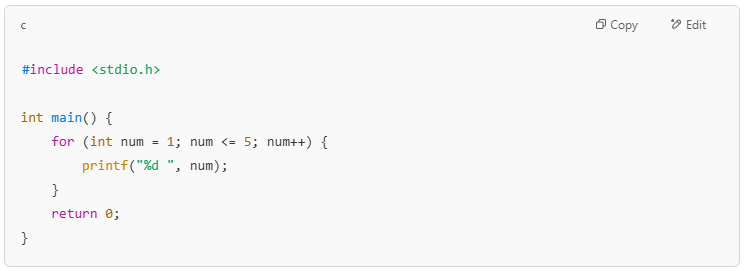
✅ This version is cleaner, structured, and easier to understand!
Thus, structured programming avoids goto
statements to improve readability and maintainability.
Key Features of Structured Programming:
✔ Uses functions to break down complex problems.
✔ Follows a top-down design approach.
✔ Enhances code readability and maintainability.
✔ Controls flow using loops and conditional statements (if, if-else, switch) instead of jumps (goto).
Modular Programming
Modular programming is an extension of structured programming that focuses on dividing a program into independent, reusable modules or functions. Each module performs a specific task and can be tested separately.
Key Features of Modular Programming:
✔ Code Reusability – Functions and modules can be reused in different programs.
✔ Easier Debugging – Each module can be tested and debugged independently.
✔ Better Collaboration – Large projects can be divided among teams.
✔ Scalability – Makes programs easier to modify and expand.
Difference Between Structured and Modular Programming
Feature | Structured Programming | Modular Programming |
---|---|---|
Approach | Uses control structures (loops, conditions) to structure code | Divides programs into smaller, reusable modules |
Code Organization | Uses functions to improve readability | Uses independent modules for better management |
Reusability | Limited to function reuse | High, as modules can be reused across different projects |
Maintainability | Easier than unstructured code | Even easier due to independent modules |
Conclusion
Both structured and modular programming help in writing efficient, maintainable, and error-free code. While structured programming focuses on logical flow, modular programming enhances code reusability and organization, making software development more scalable.