3. High-Level Language (HLL)
Definition:
High-level languages (HLL) use English-like syntax to write programs, making them easy to understand and portable across different computer architectures. They require a compiler or interpreter to convert them into machine code.
“portable” means that programs written in high-level languages (HLL) can run on different types of computer architectures without modification or with minimal changes.
Characteristics:
- Uses human-readable syntax (e.g., if-else statements, loops).
- Hardware-independent (portable across different platforms).
- High-level languages like Python, Java, or C can be compiled or interpreted to work on different hardware platforms. This is because they rely on compilers, interpreters to translate the code into machine language for the target system.
- Easier to debug and maintain.
- Debugging means finding and fixing errors (bugs) in a program to make it work correctly.
Example (Python Code for Addition):
a = 10
b = 5
sum = a + b
print(sum)
Advantages:
✔ Easy to read, write, and debug.
✔ Portable across multiple platforms.
✔ Increases programmer productivity.
“productivity” refers to how efficiently programmers can write, test, and maintain code using high-level languages (HLL).
Disadvantages:
- Slower execution compared to low-level languages (due to translation overhead).
- Why Is High-Level Language Execution Slower Than Low-Level Language (Assembly)?
Both Assembly Language and High-Level Languages require a translator:
Assembly needs an Assembler (to convert Assembly → Machine Code).
High-Level Languages need a Compiler/Interpreter (to convert High-Level Code → Machine Code).
But Assembly is Still Faster! Here’s Why:
Assembly Has a Direct 1:1 Mapping to Machine Code
Each Assembly instruction translates directly to one Machine Code instruction.
High-level languages often require multiple machine instructions for a single statement.
✅ Example: (x86 Assembly – Direct)MOV AL, 61h ; Load AL with 61h
ADD AL, 32h ; Add 32h to AL
Equivalent Machine Code (Binary)
🔹 Only 2 machine instructions!
❌ Example: (C Code – Needs Extra Steps)int a = 97;
int b = 50;
int sum = a + b;
Equivalent Machine Code (Binary)
🔹 This compiles into multiple machine instructions (variable creation, memory allocation, type checking, etc.).
🔹 This is what the CPU directly executes!
- Why Is High-Level Language Execution Slower Than Low-Level Language (Assembly)?
- Less direct hardware control.
- Assembly language “provides direct hardware control” means programmers can directly manage and interact with the CPU, memory, and hardware components without relying on an operating system. In contrast, high-level languages sacrifice direct hardware control for ease of use, portability, and abstraction, making them ideal for general-purpose programming but less suitable for system programming or performance-critical applications.
- “abstraction” in high-level languages means hiding the complexities of hardware interactions and low-level operations, allowing programmers to focus on logic rather than machine-specific details.
- Assembly language “provides direct hardware control” means programmers can directly manage and interact with the CPU, memory, and hardware components without relying on an operating system. In contrast, high-level languages sacrifice direct hardware control for ease of use, portability, and abstraction, making them ideal for general-purpose programming but less suitable for system programming or performance-critical applications.
Comparison Table
Feature | Machine Language | Assembly Language | High-Level Language |
---|---|---|---|
Ease of Use | Very difficult | Difficult | Easy |
Execution Speed | Fastest (Direct Execution) | Fast | Slower |
Hardware Dependency | Yes | Yes | No (Portable) |
Code Readability | Not human-readable | Uses mnemonics | Human-readable syntax |
Example Instruction | 10110000 01100001 | MOV AL, 61h | a = 10 |
Why is Assembly Language Hardware-Dependent?
Assembly Language is hardware-dependent because:
✅ It is a human-readable version of machine language, using mnemonics like MOV
, ADD
, etc.
✅ Each CPU architecture (x86, ARM, RISC-V, etc.) has its own unique assembly language.
✅ An x86 assembly program won’t run on an ARM processor unless rewritten.
Example:
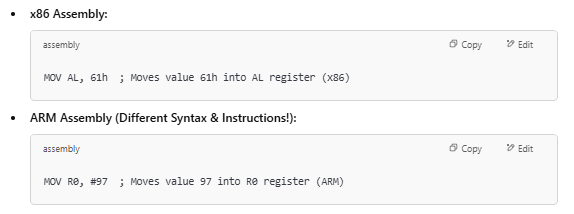
Since Assembly directly corresponds to machine code, it is specific to a CPU type, making it not portable and hardware-dependent.
Conclusion
- Machine Language: Best for direct hardware interaction, but impractical for programming.
- Assembly Language: Used for low-level programming like OS and embedded systems.
- High-Level Language: Preferred for general-purpose software development due to ease of use and portability.