Assembly Language (Low-Level Language)
Definition:
Assembly language is a symbolic representation of machine language that uses mnemonics (short, human-readable instructions). It requires an assembler to convert the code into machine language.
Characteristics:
- Uses symbolic instructions instead of binary (e.g., MOV, ADD).
- Easier to understand than machine language.
- Still hardware-dependent.
- Assembly Language is still hardware-dependent because:
- ✅ It is a human-readable version of machine language, using mnemonics like
MOV
,ADD
, etc. - ✅ Each CPU architecture (x86, ARM, RISC-V, etc.) has its own unique assembly language.
- ✅ An x86 assembly program won’t run on an ARM processor unless rewritten.
- Since Assembly directly corresponds to machine code, it is specific to a CPU type, making it not portable and still hardware-dependent.
- Portable means able to run on different hardware or operating systems without modification.
- Used in system programming (e.g., writing OS kernels, device drivers).
Example (x86 Assembly Code for Addition):
MOV AL, 61h ; Load AL register with hexadecimal value 61
ADD AL, 32h ; Add hexadecimal value 32 to AL
Advantages:
- More readable than machine language.
- Provides direct hardware control.
- “Provides direct hardware control” means programmers can directly manage and interact with the CPU, memory, and hardware components without relying on an operating system or high-level language features.
How Does Assembly Give Direct Control?
🔹 You can manipulate registers (MOV AL, 61h
moves data to a CPU register).
🔹 You can control memory addresses (e.g., direct memory access in embedded systems).
🔹 You can execute CPU-specific instructions (e.g., hardware interrupts, system calls). - Assembly gives programmers full control over hardware because:
- Machine Language is the closest to hardware (binary 0s and 1s). The CPU directly executes it.
- Assembly Language is a symbolic representation of Machine Language, using mnemonics like
MOV
,ADD
, etc.
- It allows direct control over CPU registers, memory, and hardware components.
- “Provides direct hardware control” means programmers can directly manage and interact with the CPU, memory, and hardware components without relying on an operating system or high-level language features.
- Faster execution compared to high-level languages.
- Assembly executes faster than high-level languages because:
- No Extra Translation Steps:
- High-level languages (Python, Java, C++) require a compiler or interpreter to convert code into machine language.
- Assembly is directly translated into machine code (1:1 mapping), reducing execution time.
- Example: Adding Two Numbers
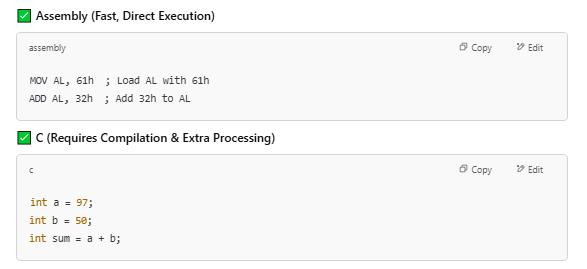
C compiler must generate multiple machine instructions for variable handling, type checking, and memory access. Assembly executes directly!
Why is Assembly Execution Faster Than High-Level Languages?
Both Assembly Language and High-Level Languages require a translator:
- Assembly needs an Assembler (to convert Assembly → Machine Code).
- High-Level Languages need a Compiler/Interpreter (to convert High-Level Code → Machine Code).
But Assembly is Still Faster! Here’s Why:
Assembly Has a Direct 1:1 Mapping to Machine Code
- Each Assembly instruction translates directly to one Machine Code instruction.
- High-level languages often require multiple machine instructions for a single statement.
✅ Example: (x86 Assembly – Direct)
MOV AL, 61h ; Load AL with 61h
ADD AL, 32h ; Add 32h to AL
Equivalent Machine Code (Binary)

🔹 Only 2 machine instructions!
❌ Example: (C Code – Needs Extra Steps)
int a = 97;
int b = 50;
int sum = a + b;
Equivalent Machine Code (Binary)
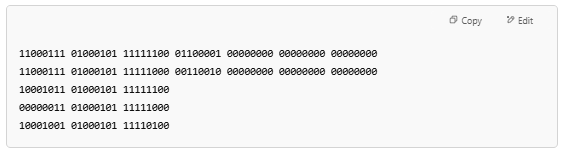
🔹 This compiles into multiple machine instructions (variable creation, memory allocation, type checking, etc.).
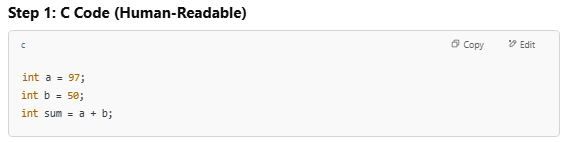
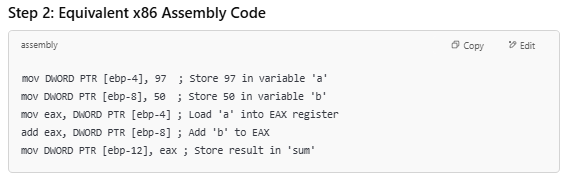
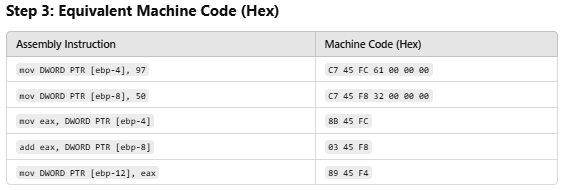
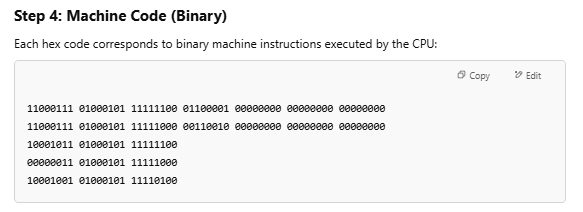
🔹 This is what the CPU directly executes!
Disadvantages:
✘ Still complex and not user-friendly.
✘ Requires knowledge of CPU architecture.
✘ Not portable (specific to a processor family).
Portable means able to run on different hardware or operating systems without modification. Since Assembly directly corresponds to machine code, it is specific to a CPU type, making it not portable and still hardware-dependent.