Different Problem-Solving Techniques in Programming
Problem-solving in programming involves various techniques to analyze, design, and implement efficient solutions. The most common methods include algorithms, pseudocodes, and flowcharts.
1. Algorithms
An algorithm is a step-by-step procedure or set of rules to solve a specific problem. It defines the logical sequence of operations that must be followed to achieve the desired result.
Types of Algorithm Techniques:
πΉ Brute Force β Try all possible solutions and choose the best one.
πΉ Divide and Conquer β Break the problem into smaller sub-problems, solve them, and combine results (e.g., Merge Sort, Quick Sort).
πΉ Greedy Algorithm β Make the best choice at each step (e.g., Dijkstraβs Algorithm).
πΉ Dynamic Programming β Solve sub-problems and store results to avoid recomputation (e.g., Fibonacci series).
Recomputation means calculating the same value multiple times unnecessarily. In Dynamic Programming, we solve sub-problems and store their results so that we donβt have to compute them again when needed later.
πΉ Backtracking β Try different solutions and backtrack when necessary (e.g., Sudoku Solver, N-Queens Problem).
Example Algorithm (Finding the Largest Number in an Array):
- Start
- Initialize a variable max to the first element of the array.
- Loop through the array and compare each element with max.
- If an element is greater than max, update max.
- After the loop ends, max holds the largest value.
- Print max and stop.
2. Pseudocode
Pseudocode is a simplified, informal way of writing algorithms using plain English and basic programming constructs. It helps programmers design solutions before actual coding.
πΉ It does not follow strict syntax like programming languages.
πΉ It focuses on logic rather than implementation details.
Basic Programming Constructs refer to the fundamental building blocks used to write programs. These include:
1οΈβ£ Sequential Execution β Code runs line by line, in order.
2οΈβ£ Variables & Data Types β Storing and manipulating values (e.g., numbers, text).
3οΈβ£ Input/Output β Taking input from users and displaying output.
4οΈβ£ Decision Making (Selection) β Using conditional statements like if-else
and switch
to control program flow.
5οΈβ£ Loops (Iteration) β Using for, while, and do-while loops to repeat tasks.
6οΈβ£ Functions (Modularity) β Breaking a program into reusable blocks of code.
Pseudocode uses these constructs in plain English, making it easier to plan and understand an algorithm before writing actual code.
Plain English means writing in a simple and easy-to-understand way, without using complex technical terms or difficult language.
For example:
πΉ Technical Language:
“Initialize an integer variable and assign it a value of 5. Subsequently, utilize a loop to iterate until the variable exceeds 10, incrementing its value by 1 in each iteration.”
πΉ Plain English:
“Start with a number 5. Keep adding 1 to it until it becomes greater than 10.”
In pseudocode, we use plain English to describe the logic of a program without worrying about syntax.
Example Pseudocode (Find the Largest Number in an Array):
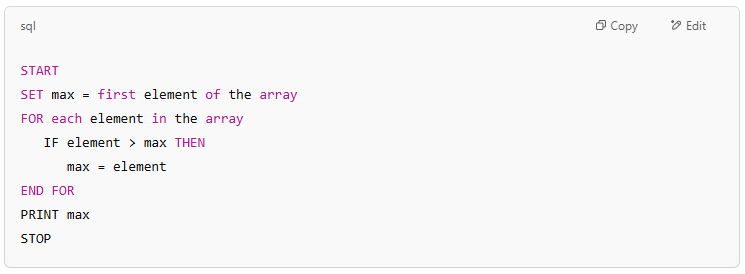
3. Flowcharts
A flowchart is a graphical representation of an algorithm. It uses different symbols to visually depict the flow of a program.
Depict means to show, illustrate, or represent something visually.
πΉ Ovals β Represent Start/Stop points.
πΉ Parallelograms β Represent Input/Output operations.
πΉ Rectangles β Represent Processing steps (calculations, assignments).
πΉ Diamonds β Represent Decision-making (if-else conditions).
Example Flowchart (Find the Largest Number in an Array):
- Start β Oval (Represents Start)
- Input Array β Parallelogram (Represents Input)
- Set max = First Element β Rectangle (Represents Processing)
- Loop through Array β Rectangle (Represents Processing)
- Compare & Update max β Diamond (Represents Decision-making, checking if the current element is greater than max)
- Print max β Parallelogram (Represents Output)
- Stop β Oval (Represents Stop)
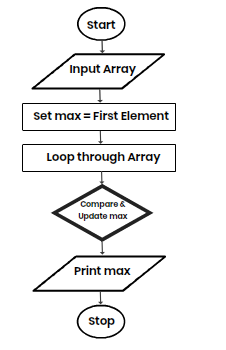
Conclusion
β
Algorithms provide structured steps for problem-solving.
β
Pseudocode helps in designing logic before actual coding.
β
Flowcharts offer a visual representation of program flow.
By combining these techniques, programmers can efficiently solve problems and develop optimized solutions.